XML DOM
The DOM (Document Object Model) defines a standard way for accessing and
manipulating documents.
The XML DOM
The XML DOM (XML Document Object Model) defines a
standard way for accessing and manipulating XML documents.
The DOM views XML documents as a tree-structure. All
elements can be accessed through the DOM tree. Their content (text and
attributes) can be modified or
deleted, and new elements can be created. The elements, their
text, and their attributes are all known as nodes.
In the examples below we use the following DOM reference to get the text from the
<to> element:
xmlDoc.getElementsByTagName("to")[0].childNodes[0].nodeValue
- xmlDoc - the XML document created by the parser.
- getElementsByTagName("to")[0] - the first <to> element
- childNodes[0] - the first child of the <to> element (the text
node)
- nodeValue - the value of the node (the text itself)
You can learn more about the XML DOM in our XML DOM
tutorial.
The HTML DOM
The HTML DOM (HTML Document Object Model) defines a
standard way for accessing and manipulating HTML documents.
All
HTML elements can be accessed through the HTML DOM.
In the examples below we use the following DOM reference to change the text of
the HTML element where id="to":
document.getElementById("to").innerHTML=
- document - the HTML document
- getElementById("to") - the HTML element where id="to"
- innerHTML - the inner text of the HTML element
You can learn more about the HTML DOM in our HTML
DOM tutorial.
Parsing an XML File - A Cross browser Example
The following code loads an XML document ("note.xml") into the XML parser:
<html>
<head>
<script type="text/javascript">
function parseXML()
{
try //Internet Explorer
{
xmlDoc=new ActiveXObject("Microsoft.XMLDOM");
}
catch(e)
{
try //Firefox, Mozilla, Opera, etc.
{
xmlDoc=document.implementation.createDocument("","",null);
}
catch(e)
{
alert(e.message);
return;
}
}
xmlDoc.async=false;
xmlDoc.load("note.xml");
document.getElementById("to").innerHTML=
xmlDoc.getElementsByTagName("to")[0].childNodes[0].nodeValue;
document.getElementById("from").innerHTML=
xmlDoc.getElementsByTagName("from")[0].childNodes[0].nodeValue;
document.getElementById("message").innerHTML=
xmlDoc.getElementsByTagName("body")[0].childNodes[0].nodeValue;
}
</script>
</head>
<body onload="parseXML()">
<h1>W3Schools Internal Note</h1>
<p><b>To:</b> <span id="to"></span><br />
<b>From:</b> <span id="from"></span><br />
<b>Message:</b> <span id="message"></span>
</p>
</body>
</html>
|
Output:
W3Schools Internal Note
To: Tove
From: Jani
Message: Don't forget me this weekend! |
Try it yourself
Important Note
To extract the text "Jani" from the XML, the syntax is:
getElementsByTagName("from")[0].childNodes[0].nodeValue
|
In the XML example there is
only one <from> tag, but you still have to specify the array index [0], because
the XML parser method getElementsByTagName() returns an array of all <from>
nodes.
Parsing an XML String - A Cross browser Example
The following code loads and parses an XML string:
<html>
<head>
<script type="text/javascript">
function parseXML()
{
text="<note>";
text=text+"<to>Tove</to>";
text=text+"<from>Jani</from>";
text=text+"<heading>Reminder</heading>";
text=text+"<body>Don't forget me this weekend!</body>";
text=text+"</note>";
try //Internet Explorer
{
xmlDoc=new ActiveXObject("Microsoft.XMLDOM");
xmlDoc.async="false";
xmlDoc.loadXML(text);
}
catch(e)
{
try // Firefox, Mozilla, Opera, etc.
{
parser=new DOMParser();
xmlDoc=parser.parseFromString(text,"text/xml");
}
catch(e)
{
alert(e.message);
return;
}
}
document.getElementById("to").innerHTML=
xmlDoc.getElementsByTagName("to")[0].childNodes[0].nodeValue;
document.getElementById("from").innerHTML=
xmlDoc.getElementsByTagName("from")[0].childNodes[0].nodeValue;
document.getElementById("message").innerHTML=
xmlDoc.getElementsByTagName("body")[0].childNodes[0].nodeValue;
}
</script>
</head>
<body onload="parseXML()">
<h1>W3Schools Internal Note</h1>
<p><b>To:</b> <span id="to"></span><br />
<b>From:</b> <span id="from"></span><br />
<b>Message:</b> <span id="message"></span>
</p>
</body>
</html>
|
Output:
W3Schools Internal Note
To: Tove
From: Jani
Message: Don't forget me this weekend! |
Try it
yourself
Note: Internet Explorer uses the loadXML() method to parse an XML
string, while other browsers uses the DOMParser object.
 |
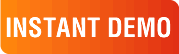
|
|
See why there are 20,000+ Ektron integrations worldwide.
Request an INSTANT DEMO or download a FREE TRIAL today. |
|
|
|