JavaScript Animation
With JavaScript we can create animated images.
Examples
Button animation
JavaScript Animation
It is possible to use JavaScript to create animated images.
The trick is to let a JavaScript change between different images on different
events.
In the following example we will add an image that should act as a link button on
a web page. We will then add an onMouseOver event and an onMouseOut event that will run
two JavaScript functions
that will change between the images.
The HTML Code
The HTML code looks like this:
<a href="http://www.w3schools.com" target="_blank">
<img border="0" alt="Visit W3Schools!"
src="b_pink.gif" name="b1"
onmouseOver="mouseOver()"
onmouseOut="mouseOut()" />
</a>
|
Note that we have given the image a name to make it possible for JavaScript
to address it later.
The onMouseOver event tells the browser that once a mouse is
rolled over the image, the browser should execute a function that will replace
the image with another image.
The onMouseOut event tells the browser that once a mouse is
rolled away from the image, another JavaScript function should be executed. This
function will insert the original image again.
The JavaScript Code
The changing between the images is done with the following JavaScript:
<script type="text/javascript">
function mouseOver()
{
document.b1.src ="b_blue.gif";
}
function mouseOut()
{
document.b1.src ="b_pink.gif";
}
</script>
|
The function mouseOver() causes the image to shift to "b_blue.gif".
The function mouseOut() causes the image to shift to "b_pink.gif".
The Entire Code
<html>
<head>
<script type="text/javascript">
function mouseOver()
{
document.b1.src ="b_blue.gif";
}
function mouseOut()
{
document.b1.src ="b_pink.gif";
}
</script>
</head>
<body>
<a href="http://www.w3schools.com" target="_blank">
<img border="0" alt="Visit W3Schools!"
src="b_pink.gif" name="b1"
onmouseOver="mouseOver()"
onmouseOut="mouseOut()" />
</a>
</body>
</html>
|
Learn XML with <oXygen/> XML Editor - Free Trial!
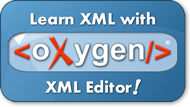 |
|
oXygen helps you learn to define,
edit, validate and transform XML documents. Supported technologies include XML Schema,
DTD, Relax NG, XSLT, XPath, XQuery, CSS.
Understand in no time how XSLT and XQuery work by using the intuitive oXygen debugger!
Do you have any XML related questions? Get free answers from the oXygen
XML forum
and from the video
demonstrations.
Download a FREE 30-day trial today!
|
|