HTML DOM Properties and Methods
Properties and methods define the programming interface to the
HTML DOM.
Programming Interface
The DOM models HTML as a set of node objects. The nodes can be accessed with
JavaScript or other programming languages. In this tutorial we use JavaScript.
The programming interface to the DOM is defined by a set standard properties
and methods.
Properties are often referred to as something that is (i.e. nodename
is "p").
Methods are often referred to as something that is done (i.e. delete
"p").
HTML DOM Properties
These are some typical DOM properties:
- x.innerHTML - the inner text value of x (a HTML element)
- x.nodeName - the name of x
- x.nodeValue - the value of x
- x.parentNode - the parent node of x
- x.childNodes - the child nodes of x
- x.attributes - the attributes nodes of x
Note: In the list above, x is a node object (HTML element).
HTML DOM Methods
- x.getElementById(id) - get the element with a specified id
- x.getElementsByTagName(name) - get all elements with a specified
tag name
- x.appendChild(node) - insert a child node to x
- x.removeChild(node) - remove a child node from x
Note: In the list above, x is a node object (HTML element).
innerHTML
The easiest way to get or modify the content of an element is by using the
innerHTML property.
innerHTML is not a part of the W3C DOM specification. However, it is
supported by all major browsers.
The innerHTML property is useful for returning or replacing the content of
HTML elements (including <html> and <body>), it can also be used to view the
source of a page that has been dynamically modified.
Example
The JavaScript code to get the text from a <p> element with the id "intro" in
a HTML document:
txt=document.getElementById("intro").innerHTML
After the execution of the statement, txt will hold the value "Hello World!"
Explained:
- document - the current HTML document
- getElementById("intro") - the <p> element with the id "intro"
- innerHTML - the inner text of the HTML element
In the example above, getElementById is a method, while innerHTML is a property.
Try it
yourself
childNodes and nodeValue
The W3C DOM specified way of getting to the content of an element works like
this:
The JavaScript code to get the text from a <p> element with the id "intro" in
a HTML document:
txt=document.getElementById("intro").childNodes[0].nodeValue
After the execution of the statement, txt will hold the value "Hello World!"
Explained:
- document - the current HTML document
- getElementById("intro") - the <p> element with the id "intro"
- childNodes[0] - the first child of the <p> element (the text
node)
- nodeValue - the value of the node (the text itself)
In the example above, getElementById is a method, while childNodes and
nodeValue are properties.
Try it
yourself
In this tutorial we will mostly use the innerHTML property. However, learning
the method above is useful for understanding the tree structure of the DOM and
dealing with XML files.
 |
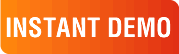
|
|
See why there are 20,000+ Ektron integrations worldwide.
Request an INSTANT DEMO or download a FREE TRIAL today. |
|
|
|