VBScript Conditional Statements
Examples
The if...then...else statement
This example demonstrates how to write the if...then..else statement.
The if...then...elseif... statement
This example demonstrates how to write the if...then...elseif statement.
The select case statement
This example demonstrates how to write the select case statement.
Conditional Statements
Very often when you write code, you want to perform different actions for different decisions.
You can use conditional statements in your code to do this.
In VBScript we have four conditional statements:
- if statement - use this statement if you want to execute a set of
code when a condition is true
- if...then...else statement - use this statement if you want to
select one of two sets of lines to execute
- if...then...elseif statement - use this statement if you want to
select one of many sets of lines to execute
- select case statement - use this statement if you want to select one of many sets
of lines to execute
If....Then.....Else
You should use the If...Then...Else statement if you want to
- execute some code if a
condition is true
- select one of two blocks of code to execute
If you want to execute only one statement when a condition is true, you
can write the code on one line:
if i=10 Then msgbox "Hello"
|
There is no ..else.. in this syntax. You just tell the code to
perform one action if the condition is true (in this case if i=10).
If you want to execute more than one statement when a condition is true,
you must put each statement on separate lines and end the statement with the
keyword "End If":
if i=10 Then
msgbox "Hello"
i = i+1
end If
|
There is no ..else.. in this syntax either. You just tell the
code to perform multiple actions if the condition is true.
If you want to execute a statement if a condition is true and execute another
statement if the condition is not true, you must add the "Else"
keyword:
if i=10 then
msgbox "Hello"
else
msgbox "Goodbye"
end If
|
The first block of code will be executed if the condition is true, and the other block
will be executed otherwise (if i is not equal to 10).
If....Then.....Elseif
You can use the if...then...elseif statement if you want to select one of many blocks of
code to execute:
if payment="Cash" then
msgbox "You are going to pay cash!"
elseif payment="Visa" then
msgbox "You are going to pay with visa."
elseif payment="AmEx" then
msgbox "You are going to pay with American Express."
else
msgbox "Unknown method of payment."
end If
|
Select Case
You can also use the SELECT statement if you want to select one of many blocks of
code to execute:
select case payment
case "Cash"
msgbox "You are going to pay cash"
case "Visa"
msgbox "You are going to pay with visa"
case "AmEx"
msgbox "You are going to pay with American Express"
case Else
msgbox "Unknown method of payment"
end select
|
This is how it works: First we have a single expression (most often a
variable), that is evaluated once. The value of the expression is then compared with the
values for each Case in the structure. If there is a match, the block of code
associated with that Case is executed.
Learn XML with <oXygen/> XML Editor - Free Trial!
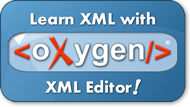 |
|
oXygen helps you learn to define,
edit, validate and transform XML documents. Supported technologies include XML Schema,
DTD, Relax NG, XSLT, XPath, XQuery, CSS.
Understand in no time how XSLT and XQuery work by using the intuitive oXygen debugger!
Do you have any XML related questions? Get free answers from the oXygen
XML forum
and from the video
demonstrations.
Download a FREE 30-day trial today!
|
|