PHP str_ireplace() Function
Complete PHP String Reference
Definition and Usage
The str_ireplace() function replaces some characters with some other
characters in a string.
This function works by the following rules:
- If the string to be searched is an array, it returns an array
- If the string to be searched is an array, find and replace is performed with every
array element
- If both find and replace are arrays, and replace has fewer elements than
find, an empty string will be used as replace
- If find is an array and replace is a string, the replace string will
be used for every find value
Syntax
str_ireplace(find,replace,string,count)
|
Parameter |
Description |
find |
Required. Specifies the value to find |
replace |
Required. Specifies the value to replace the value in
find |
string |
Required. Specifies the string to be searched |
count |
Optional. A variable that counts the number of replacements |
Tips and Notes
Note: This function is case-insensitive. Use str_replace() to perform
a case-sensitive search.
Note: This function is binary-safe.
Example 1
<?php
echo str_ireplace("WORLD","Peter","Hello world!");
?>
|
The output of the code above will be:
Example 2
In this example we will demonstrate str_ireplace() with an array and a count
variable:
<?php
$arr = array("blue","red","green","yellow");
print_r(str_ireplace("RED","pink",$arr,$i));
echo "Replacements: $i";
?>
|
The output of the code above will be:
Array
(
[0] => blue
[1] => pink
[2] => green
[3] => yellow
)
Replacements: 1
|
Example 3
In this example we will demonstrate str_ireplace() with
less elements in replace than find:
<?php
$find = array("HELLO","WORLD");
$replace = array("B");
$arr = array("Hello","world","!");
print_r(str_ireplace($find,$replace,$arr));
?>
|
The output of the code above will be:
Array
(
[0] => B
[1] =>
[2] => !
)
|
Complete PHP String Reference
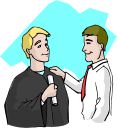 |
|
Get Your Diploma!
W3Schools' Online Certification Program is the perfect solution for busy
professionals who need to balance work, family, and career building.
The HTML Certificate is for developers who want to document their knowledge of HTML, XHTML, and CSS.
The JavaScript Certificate is for developers who want to document their knowledge of JavaScript and the HTML DOM.
The XML Certificate is for developers who want to document their knowledge of XML, XML DOM and XSLT.
The ASP Certificate is for developers who want to document their knowledge of ASP, SQL, and ADO.
The PHP Certificate is for developers who want to document their knowledge of PHP and SQL (MySQL).
|
|