PHP mysql_real_escape_string() Function
Complete PHP MySQL Reference
Definition and Usage
The mysql_real_escape_string() function escapes special characters in a
string for use in an SQL statement
The following characters are affected:
This function returns the escaped string on success, or FALSE on
failure.
Syntax
mysql_real_escape_string(string,connection)
|
Parameter |
Description |
string |
Required. Specifies the string to be escaped |
connection |
Optional. Specifies the MySQL connection. If not specified,
the last connection opened by mysql_connect() or mysql_pconnect() is used. |
Tips and Notes
Note: Use this function to prevent database attack!
Example 1
<?php
$con = mysql_connect("localhost", "peter", "abc123");
if (!$con)
{
die('Could not connect: ' . mysql_error());
}
// some code to get username and password
// escape username and password for use in SQL
$user = mysql_real_escape_string($user);
$pwd = mysql_real_escape_string($pwd);
$sql = "SELECT * FROM users WHERE
user='" . $user . "' AND password='" . $pwd . "'"
// more code
mysql_close($con);
?>
|
Example 2
Database attack. This example demonstrates what could happen if we do not use
the mysql_real_escape_string() function on the username and password:
<?php
$con = mysql_connect("localhost", "peter", "abc123");
if (!$con)
{
die('Could not connect: ' . mysql_error());
}
$sql = "SELECT * FROM users
WHERE user='{$_POST['user']}'
AND password='{$_POST['pwd']}'";
mysql_query($sql);
// We didn't check username and password.
// Could be anything the user wanted! Example:
$_POST['user'] = 'john';
$_POST['pwd'] = "' OR ''='";
// some code
mysql_close($con);
?>
|
The SQL sent would be:
SELECT * FROM users
WHERE user='john' AND password='' OR ''=''
|
This means that anyone could log in without a valid password!
Example 3
The correct way to do it to prevent database attack:
<?php
function check_input($value)
{
// Stripslashes
if (get_magic_quotes_gpc())
{
$value = stripslashes($value);
}
// Quote if not a number
if (!is_numeric($value))
{
$value = "'" . mysql_real_escape_string($value) . "'";
}
return $value;
}
$con = mysql_connect("localhost", "peter", "abc123");
if (!$con)
{
die('Could not connect: ' . mysql_error());
}
// Make a safe SQL
$user = check_input($_POST['user']);
$pwd = check_input($_POST['pwd']);
$sql = "SELECT * FROM users WHERE
user=$user AND password=$pwd";
mysql_query($sql);
mysql_close($con);
?>
|
Complete PHP MySQL Reference
Learn how your website performs under various load conditions
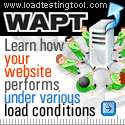 |
|
WAPT
is a load, stress and performance testing tool for websites and web-based applications.
In contrast to "800-pound gorilla" load testing tools, it is designed to minimize the learning
curve and give you an ability to create a heavy load from a regular workstation.
WAPT is able to generate up to 3000 simultaneously acting virtual users using standard hardware configuration.
Virtual users in each profile are fully customizable. Basic and NTLM authentication methods are supported.
Graphs and reports are shown in real-time at different levels of detail, thus helping to manage the testing process.
Download the free 30-day trial!
|
|