PHP extract() Function
Complete PHP Array Reference
Definition and Usage
The extract() function imports variables into the local symbol table from an
array.
This function uses array keys as variable names and values as variable
values. For each element it will create a variable in the current symbol table.
This function returns the number of variables extracted on success.
Syntax
extract(array,extract_rules,prefix)
|
Parameter |
Description |
array |
Required. Specifies the array to use |
extract_rules |
Optional. The extract() function checks for invalid
variable names and collisions with existing variable names. This parameter
specifies how invalid and colliding names are treated. Possible values:
- EXTR_OVERWRITE - Default. On collision, the existing variable is
overwritten
- EXTR_SKIP - On collision, the existing variable is not overwritten
- EXTR_PREFIX_SAME - On collision, the variable name will be given a
prefix
- EXTR_PREFIX_ALL - All variable names will be given a prefix
- EXTR_PREFIX_INVALID - Only invalid or numeric variable names will be
given a prefix
- EXTR_IF_EXISTS - Only overwrite existing variables in the current
symbol table, otherwise do nothing
- EXTR_PREFIX_IF_EXISTS - Only add prefix to variables if the same
variable exists in the current symbol table
- EXTR_REFS - Extracts variables as references. The imported variables
are still referencing the values of the array parameter
|
prefix |
Optional. If EXTR_PREFIX_SAME, EXTR_PREFIX_ALL,
EXTR_PREFIX_INVALID or EXTR_PREFIX_IF_EXISTS are used in the extract_rules
parameter, a specified prefix is required.
This parameter specifies the prefix. The prefix is automatically
separated from the array key by an underscore character. |
Example 1
<?php
$a = 'Original';
$my_array = array("a" => "Cat","b" => "Dog", "c" => "Horse");
extract($my_array);
echo "\$a = $a; \$b = $b; \$c = $c";
?>
|
The output of the code above will be:
$a = Cat; $b = Dog; $c = Horse
|
Example 2
With all parameters in use:
<?php
$a = 'Original';
$my_array = array("a" => "Cat","b" => "Dog", "c" => "Horse");
extract($my_array, EXTR_PREFIX_SAME, 'dup');
echo "\$a = $a; \$b = $b; \$c = $c; \$dup_a = $dup_a;";
?>
|
The output of the code above will be:
$a = Original; $b = Dog; $c = Horse; $dup_a = Cat;
|
Complete PHP Array Reference
Learn XML with <oXygen/> XML Editor - Free Trial!
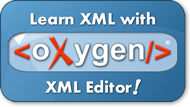 |
|
oXygen helps you learn to define,
edit, validate and transform XML documents. Supported technologies include XML Schema,
DTD, Relax NG, XSLT, XPath, XQuery, CSS.
Understand in no time how XSLT and XQuery work by using the intuitive oXygen debugger!
Do you have any XML related questions? Get free answers from the oXygen
XML forum
and from the video
demonstrations.
Download a FREE 30-day trial today!
|
|