JavaScript replace() Method
Complete String Object Reference
Definition and Usage
The replace() method is used to replace some characters with some other characters in a
string.
This method supports regular expressions. You can learn about the
RegExp object in our JavaScript tutorial.
Syntax
stringObject.replace(findstring,newstring)
|
Parameter |
Description |
findstring |
Required. Specifies a string value to find. To perform a
global search add a 'g' flag to this parameter and to perform a
case-insensitive search add an 'i' flag |
newstring |
Required. Specifies the string to replace the found value from findstring |
Tips and Notes
Note: The replace() method is case sensitive.
Example 1 - Standard Replace
In the following example we will replace the word Microsoft with W3Schools:
<script type="text/javascript">
var str="Visit Microsoft!";
document.write(str.replace(/Microsoft/, "W3Schools"));
</script>
|
The output of the code above will be:
Note: In the following example the word Microsoft will not be replaced
(because the replace() method is case sensitive):
<script type="text/javascript">
var str="Visit Microsoft!";
document.write(str.replace(/microsoft/, "W3Schools"));
</script>
|
The output of the code above will be:
Example 2 - Case-insensitive Search
In the following example we will perform a case-insensitive search, and the word Microsoft
will be replaced:
<script type="text/javascript">
var str="Visit Microsoft!";
document.write(str.replace(/microsoft/i, "W3Schools"));
</script>
|
The output of the code above will be:
Example 3 - Global Search
In the following example we will perform a global match, and the word Microsoft
will be replaced each time it is found:
<script type="text/javascript">
var str="Welcome to Microsoft! ";
str=str + "We are proud to announce that Microsoft has ";
str=str + "one of the largest Web Developers sites in the world.";
document.write(str.replace(/Microsoft/g, "W3Schools"));
</script>
|
The output of the code above will be:
Welcome to W3Schools! We are proud to announce that W3Schools
has one of the largest Web Developers sites in the world.
|
Example 4 - Global and Case-insensitive Search
In the following example we will perform a global and case-insensitive match,
and the word Microsoft will be replaced each time it is found, independent of
upper and lower case characters:
<script type="text/javascript">
var str="Welcome to Microsoft! ";
str=str + "We are proud to announce that Microsoft has ";
str=str + "one of the largest Web Developers sites in the world.";
document.write(str.replace(/microsoft/gi, "W3Schools"));
</script>
|
The output of the code above will be:
Welcome to W3Schools! We are proud to announce that W3Schools
has one of the largest Web Developers sites in the world.
|
Try-It-Yourself Demos
replace()
How to use replace() to replace some characters in a string.
replace()
Case-insensitive search
How to use replace() with the 'i' flag to replace some characters in a string.
replace()
Global search
How to use replace() with the 'g' flag to replace some characters in a string.
Complete String Object Reference
 |
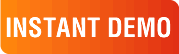
|
|
See why there are 20,000+ Ektron integrations worldwide.
Request an INSTANT DEMO or download a FREE TRIAL today. |
|
|
|